一.使用Ribbon实现客户端负载均衡(默认采用的是轮询方式)
0.ribbon的依赖在eureka中已经包含了,所以不用单独导入相关的依赖
1.将restTemplate加上@LoadBalanced注解
@SpringBootApplication
@EnableEurekaClient //标注App此应用为Eureka的客户端服务
@MapperScan(value = {"com.ooyhao.cloud.serverrole.mapper"})
public class ServerRoleApplication {
public static void main(String[] args) {
SpringApplication.run(ServerRoleApplication.class, args);
}
/**
* 实例化ribbon使用的RestTemplate
* @return
*/
@Bean
@LoadBalanced //表示使用负载均衡的方式进行
public RestTemplate restTemplate(){
return new RestTemplate();
}
}
2.在controller进行 服务调用的时候,可以直接使用服务名称(SERVER-USER)
/**
* getForObject: get 表示为Get请求,Object表示为返回值是一个对象。
* 参数说明:
* 1.http请求访问地址
* 2.返回的Object(对象)的类型
* 3.参数
* @param id
* @return
*/
@RequestMapping("/user/{id}")
public List<User> findUsersByRoleId(@PathVariable("id") Integer id){
for(int i =0; i < 20; i++){
InstanceInfo instanceInfo = eurekaClient.getNextServerFromEureka("SERVER-USER", false);
String homePageUrl = instanceInfo.getHomePageUrl();
System.out.println(homePageUrl);
List<User> user = restTemplate.getForObject(
"http://SERVER-USER/user/findUsersByRoleId/{id}",
List.class,
id);
}
return null;
}
二、使用Feign进行声明式服务调用
0.在pom文件中导入相关的依赖
<!--Feign声明式服务调用-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-feign</artifactId>
<version>1.4.0.RELEASE</version>
</dependency>
1.在主程序类上添加@EnableFeignClients注解,标记为Feign的客户端。
@SpringBootApplication
@EnableEurekaClient //标注App此应用为Eureka的客户端服务
@EnableFeignClients
@MapperScan(value = {"com.ooyhao.cloud.serverrole.mapper"})
public class ServerRoleApplication {
public static void main(String[] args) {
SpringApplication.run(ServerRoleApplication.class, args);
}
}
2. 定义一个接口,通过@FeignClient注解来标记是使用哪个服务。然后就是写Controller一样,
通过 @FeignClient 注解指定服务名来绑定服务,然后再使用 Spring MVC 的注解来绑定具体该服务提供的 REST 接口
@FeignClient(value = "SERVER-USER")
public interface UserService {
@RequestMapping(value = "/user/findUsersByRoleId/{id}")
List<User> findUserByRoleId(@PathVariable("id") Integer id);
}
3.在需要调用的地方使用UserService即可。
在开始介绍 Spring Cloud Feign 的参数绑定之前,先扩展一下服务提供方 hello-service 。增加下面这些接口定义,其中包含带有 Request 参数的请求、带有 Header 信息的请求、带有 RequestBody 的请求以及请求响应体中是一个对象的请求。
package com.example.demo.web;
import org.apache.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cloud.client.ServiceInstance;
import org.springframework.cloud.client.discovery.DiscoveryClient;
import org.springframework.web.bind.annotation.*;
import java.util.Random;
/**
* @author lxx
* @version V1.0.0
* @date 2017-8-9
*/
@RestController
public class HelloController {
private final Logger logger = Logger.getLogger(getClass());
@Autowired
private DiscoveryClient client;
@RequestMapping(value = "/index")
public String index(){
ServiceInstance instance = client.getLocalServiceInstance();
// 让处理线程等待几秒钟
int sleepTime = new Random().nextInt(3000);
logger.info("sleepTime:"+sleepTime);
try {
Thread.sleep(sleepTime);
} catch (InterruptedException e) {
e.printStackTrace();
}
logger.info("/hello:host:"+instance.getHost()+" port:"+instance.getPort()
+" service_id:"+instance.getServiceId());
return "hello world!";
}
@RequestMapping(value = "/hello1", method = RequestMethod.GET)
public String hello1(@RequestParam String name){
return "HELLO " + name;
}
@RequestMapping(value = "/hello2", method = RequestMethod.GET)
public User hello2(@RequestHeader String name, @RequestHeader Integer age){
return new User(name, age);
}
@RequestMapping(value = "/hello3", method = RequestMethod.POST)
public String hello3(@RequestBody User user){
return "HELLO," + user.getName()+","+user.getAge();
}
}
注意:在参数绑定时,@RequestParam、@RequestHeader等可以指定参数名称的注解,他们的 value 不能少。在 Spring MVC 中,这些注解会根据参数名来作为默认值,但是在 Feign 中绑定参数必须通过 value 属性来指明具体的参数名,不然会抛出异常 IllegalStateException ,value 属性不能为空。
------------------------------------------------
关注微信公众号获取更多资源
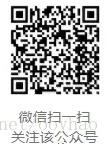